19. While 和 For 循环
Python vs. C++ While
下面,你会看到一个 Python while 循环与 C++ while循环对比的例子。它们非常相似!
该示例以 elapsed_time 变量中的整数 15 开始。每次迭代,整数减 1。一旦 elapsed_time 达到 0,程序即离开 while 循环。
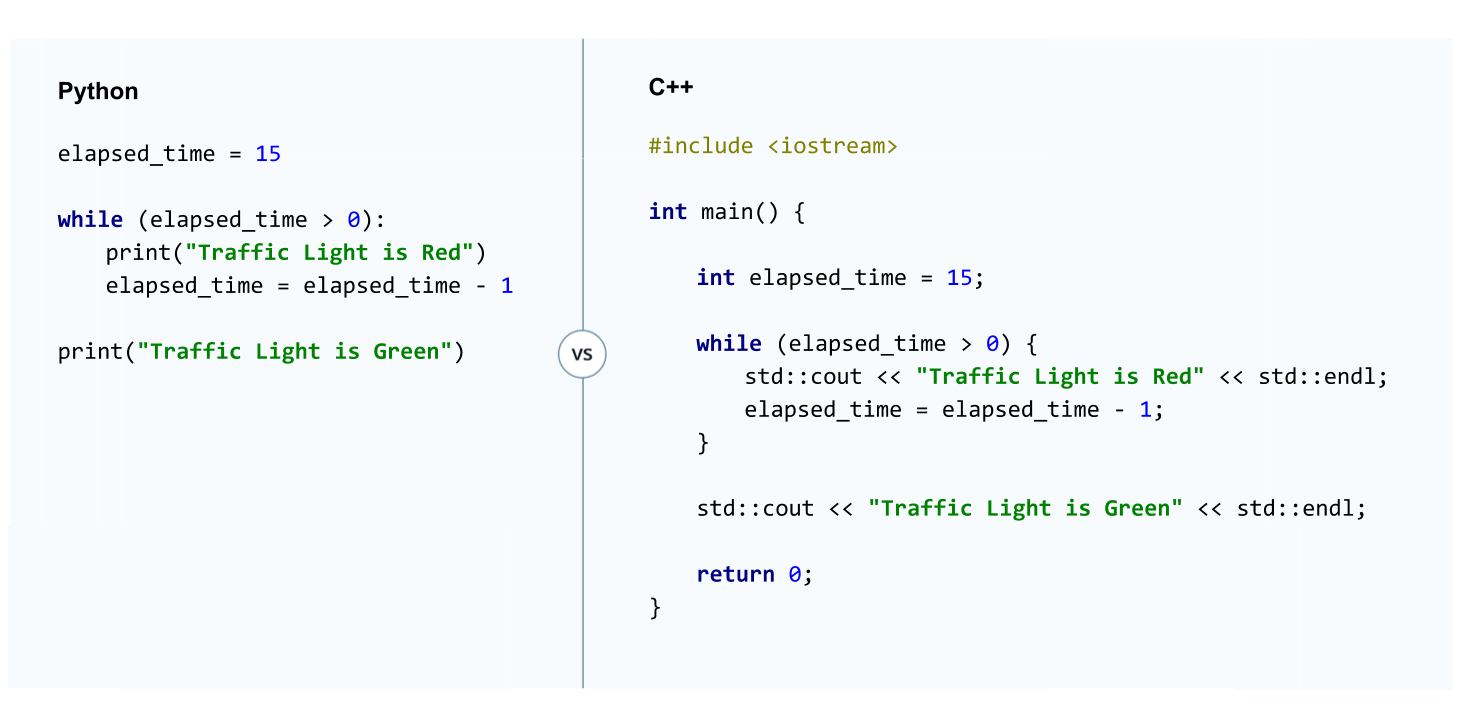
普通的 while 语句是这样的:
while (<some criteria>) {
statement_1;
statement_2;
statement_3;
....etc
}
Python vs. C++ For 循环
for 循环语法在 Python 和 C++ 中也非常相似。
下面的例子很像 while 循环,区别在于 count 变量在增加而不是减少。
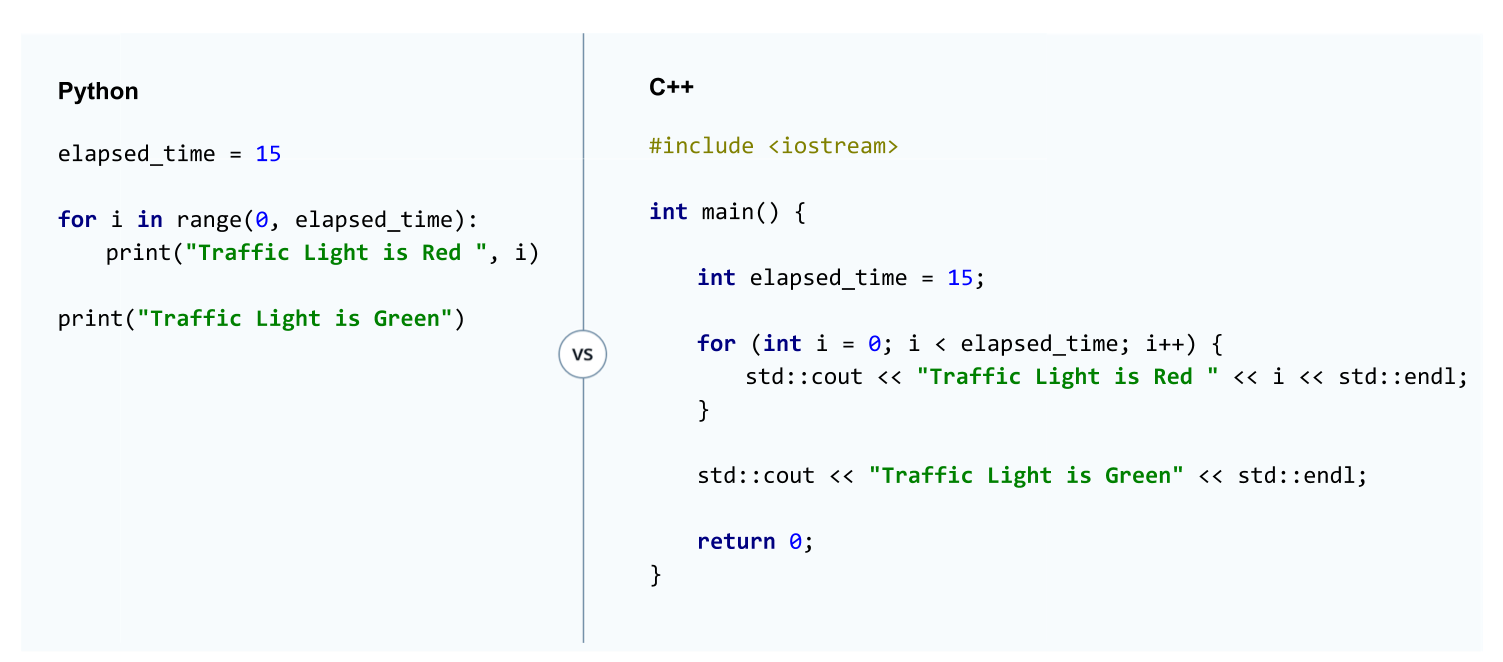
有一点需要注意的是,Python 如何迭代 i 变量,以及 C++ 如何迭代。
对于 Python,迭代器是这样定义的:
i in range(0, elapsed_time)
Python 的 range() 函数生成一个数字列表,在本例中,列表为
[0,1,2,3,4,5,6,7,8,9,10,11,12,13,14]
然后,Python 将这些值依次分配给 i 变量,直到列表末尾。
对于 C++,迭代代码为:
(int i = 0; i < elapsed_time; i++)
首先,声明变量 i 并分配一个值(在本例中为零)。然后,for 循环检查 if
i < elapsed_time
如果为 true,那么 i 将增加 1,for 循环中的代码继续运行。
当
i =14
时,for 循环最后一次运行。代码检查确定 14 小于 15,将 i 增加到 15,并在循环中运行代码。然后代码检查 15 是否小于 15。结果为 false,因此 for 循环不再运行。
游乐场 - for 循环
本游乐场专门针对 for 循环编程。注释中,我们有一个上手建议,你可以对比你的代码与 solution.cpp 中的参考答案。
Start Quiz:
#include <iostream>
int main() {
//TODO: Use this as a playground to write a for loop and if statements
// in the same program.
// For example, write a for loop that iterates from 0 to 80.
// If the iterator is greater than or equal to 0 but less than 10,
// output the phrase 'slow'
// If the iterator is between 10 inclusive
// and less than 30, output the phrase 'medium'
// If the iterator is between 30 inclusive and 70, output the phrase 'fast'
// If the iterator is greater than 70 inclusive, output the phrase 'too fast'
return 0;
}
#include <iostream>
int main() {
for (int i = 0; i < 80; i++) {
if (i < 10) {
std::cout << "slow" << std::endl;
}
else if (i < 30) {
std::cout << "medium" << std::endl;
}
else if (i < 70) {
std::cout << "fast" << std::endl;
}
else {
std::cout << "too fast" << std::endl;
}
}
return 0;
}